Plug & Play SMS Integration
Version 3.7.2 introduces the Plug & Play SMS Integration mechanism, which allows integration of external SMS service providers with the Veridium Server.
How to use and handle a Custom SMS Service Provider?
To be able to use a custom SMS service provider, the following contract must be implemented:
public interface ISmsService {
/**
* Initializes the SMS Service with the given configuration
*
* @param configuration A map containing key-value pairs for configuration
* @param trustManagerFactory The Trust Store provided by Veridium
*/
void init(Map<String, String> configuration, TrustManagerFactory trustManagerFactory);
/**
* Sends SMS using the provided SmsBody (using Veridium templates)
*
* @param smsBody The SMS Body Object
* @return true if the SMS was sent successfully, false otherwise
*/
boolean sendSms(SmsBody smsBody);
/**
* Sends SMS with context using the provided SmsContext
*
* @param smsContext The SMS Context object
* @return true if the SMS was sent successfully, false otherwise
*/
boolean sendSmsWithContext(SmsContext smsContext);
}
public class SmsBody {
/**
* Represents the phone number associated with the SMS message.
* It holds the recipient's phone number.
*/
private String phoneNumber;
/**
* Represents the content of the SMS message.
* This variable stores the actual text or body of the message.
*/
private String message;
public SmsBody(String phoneNumber, String message) {
this.phoneNumber = phoneNumber;
this.message = message;
}
/**
* Represents the phone number associated with the SMS message.
* It holds the recipient's phone number.
*/
private String phoneNumber;
/**
* Represents the name of the context associated with the SMS message.
* It acts as an identifier or label for the specific context in which
* the SMS is being used.
*/
private String contextName;
/**
* Represents a collection of parameters associated with the SMS context.
* The map contains key-value pairs where both the key and the value are strings.
* The parameters store the generated values depending on the context.
*/
private Map<String, String> parameters;
public SmsContext(String phoneNumber, String contextName, Map<String, String> parameters) {
this.phoneNumber = phoneNumber;
this.contextName = contextName;
this.parameters = parameters;
}
How to handle the contract dependency?
The contract is published as artifact in Nexus, and it is available for use as a dependency.
Example for Maven:
<dependency>
<groupId>com.veridiumid</groupId>
<artifactId>integration-custom-sms-contract</artifactId>
<version>1.0.0</version>
</dependency>
Example for Gradle:
implementation 'com.veridiumid:integration-custom-sms-contract:1.0.0'
There will be 2 ways of generating SMSs:
1. Using Veridium Templates:
In this case Veridium system will handle and generate the sms body based on the existing templates in Settings / Messaging / SMS Templates:
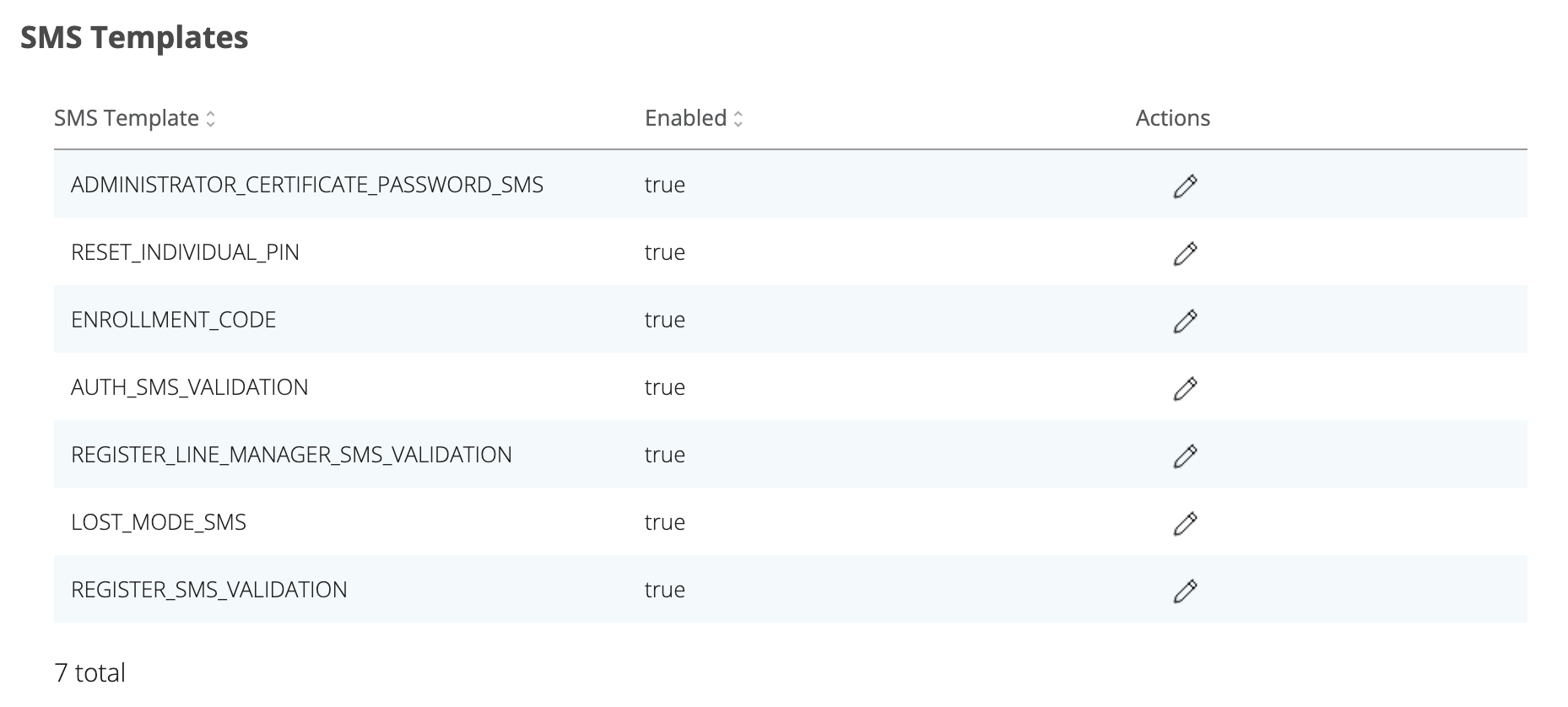
System workflow:
Veridium system processes the template:
Replace placeholders in the body template.
Example for LOST_MODE_SMS use case: “The lost mode code is ABCD1234. The code is valid 2 hours.”
Generate the object for the customer:

Pass the object to the customer’s implementation:
2. Using Customer Templates:
In this case the customer must handle and generate the sms body based on the Context and Parameters sent by Veridium system.
Overview of existing Use Cases and the parameters associated with them:
Context: LOST_MODE_SMS
Description:
Used when the Lost Mode is activated.
Parameters:
validationCode: a generated code for “lost mode”
remainingTime: how much time this code remains valid
Context: RESET_INDIVIDUAL_PIN
Description:
Used when the individual PIN needs to be reset.
Parameters:
validationCode: the newly generated PIN (one-time use)
Context: AUTH_SMS_VALIDATION
Description:
Used when the user wants to authenticate using the SMS approach.
Parameters:
validationCode: a code for user authentication or second-factor login
Context: REGISTER_LINE_MANAGER_SMS_VALIDATION
Description:
Used when enrolling the SMS authenticator and the message is sent to the Line Manager.
Parameters:
memberName: the name of the team member requesting registration
validationCode: the code for manager authorisation
Context: REGISTER_SMS_VALIDATION
Description:
Used when the user account must be enrolled.
Parameters:
validationCode: the one-time code to confirm registration
Context: ADMINISTRATOR_CERTIFICATE_PASSWORD_SMS
Description:
Used to send the certificate password via SMS to the mobile phone associated with the administrator account.
Parameters:
certificatePassword: the password for certificate
Context: ENROLLMENT_CODE
Description:
Used to send the validation code for enrollment.
Parameters:
code: the enrollment code
numberOfUsages: how many times the code can be used
remainingTimeInMinutes: expiration timeframe in minutes
System workflow:
Scenario: LOST_MODE_SMS
Purpose: used when a user activates “Lost Mode” and requires a validation code
Input object: SmsContext
scenario: “LOST_MODE_SMS”
phoneNumber: the recipient’s phone number
parameters:
validationCode: the validation code for lost mode
remainingTime: the number of hours the code remains valid
Expected behaviour:
The customer will implement send(SmsContext smsContext) method.
In the send(SmsContext smsContext) method the customer should:
Check if the scenario exists on their end.
Use the provided parameters to build and send its own message.
Example message:
Parameters:
validationCode = “ABCD9871”
remainingTime = “1”
Message: “The lost mode code is ABCD9871. The code is valid 1 hour.”
Deployment:
After implementing the contract, the custom implementation must be packaged into a JAR file.
Ensure the JAR file is added to the correct location, meaning:
The jar should be copied to each veridium WEBAPP server, in this location "/opt/veridiumid/tomcat/webapps/websec/WEB-INF/lib/".
The owner of the file should be veridiumid user: chwon ver_tomcat.veridiumid "/opt/veridiumid/tomcat/webapps/websec/WEB-INF/lib/newJar.jar"
Use the Custom SMS Service Provider:
A Tomcat restart is required to load newly added lib. ( systemctl restart ver_tomcat )
Check if the service was restarted successfully: "/etc/veridiumid/scripts/check_services.sh"
The logs are in this location:
less "/var/log/veridiumid/tomcat/catalina.out"
less "/var/log/veridiumid/tomcat/bops.log"
The SMS manager will dynamically detect and load the custom implementation.
To configure this Custom SMS Service Provider from Admin the next steps must be followed:
Go to Settings / Messaging / SMS section and go to Custom Sms Service Integration:
Add the configuration of the SMS Client that has been implemented. The <key, value> pattern must be used, otherwise the configuration cannot be saved.
NOTE: Make sure that the <key,value> pairs exists and are valid, to avoid runtime exceptions during initialisation of the SMS Service!
Add the absolute path to the JAR file of SMS Service Provider. (eg.: /Users/work/veridiumid-server/projects/integrations/integration-custom-sms-service-provider/build/libs/integration-custom-sms-service-provider.jar!/com/veridiumid/customsmsserviceprovider/client/TwilioHttpClient.class)
NOTE: The absolute path must contain ‘!' after jar’s name!If you want to Use Veridium Templates click on the switch button, otherwise the templates provided by the new SMS service provider will be used.
d. Save the changes:
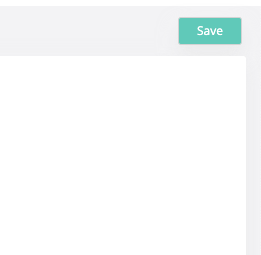
Click on Use Custom Sms Service switch button.
IMPORTANT NOTE: Deactivate the other ways of sending SMSs (Twilio & Use Mock Sms Service) in case they are active, otherwise Twilio will be used, as it is the default SMS service provider.
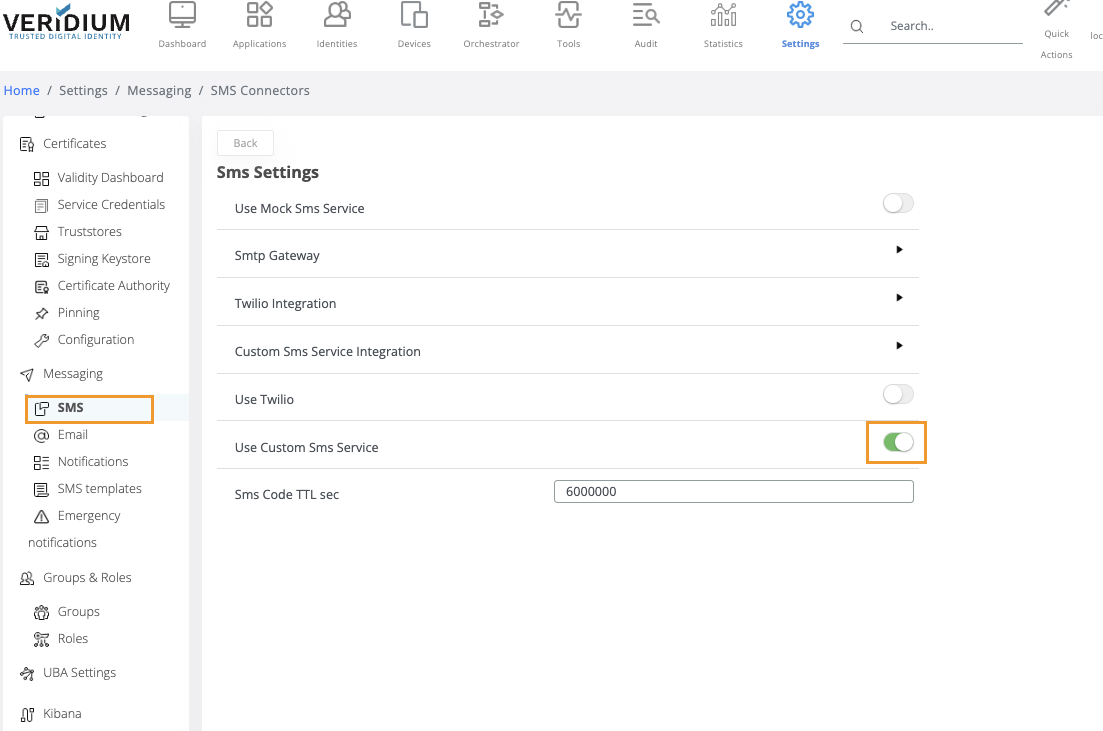
Send a test SMS:
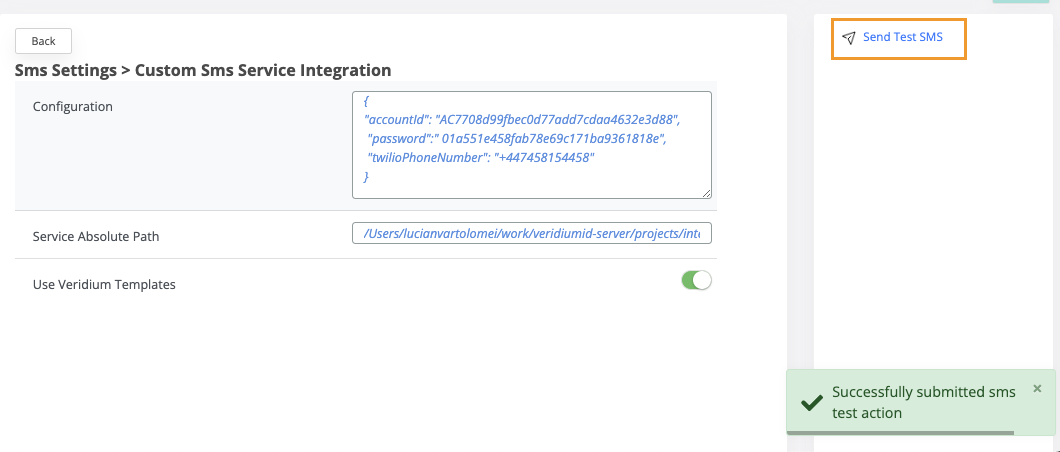
Error when sending the SMS:
When this error is displayed it means that the SMS service could not be initialised. The Configuration and Service Absolute Path must be double-checked.